Creating an ERC-20 Token: A Beginner’s Guide
- Krypto Hippo
- Feb 21
- 6 min read
Table of Contents
Introduction: What is an ERC-20 Token?
Why Create an ERC-20 Token?
Prerequisites for Creating an ERC-20 Token
A. Understanding Smart Contracts
B. Basic Knowledge of Solidity
Step-by-Step Guide to Creating an ERC-20 Token
A. Setting Up the Development Environment
B. Writing the Smart Contract
C. Deploying the Smart Contract
ERC-20 Token Functions Explained
How to Interact with Your ERC-20 Token
Best Practices for Creating a Secure ERC-20 Token
Token Audits and Security Considerations
Real-World Applications of ERC-20 Tokens
Conclusion: Start Your Token Journey Today
FAQ
1. Introduction: What is an ERC-20 Token?
In the world of cryptocurrency, ERC-20 tokens are some of the most widely used and recognized assets. But what exactly is an ERC-20 token, and why should you consider creating one?
An ERC-20 token is a type of cryptocurrency token that is built on the Ethereum blockchain. The term "ERC-20" stands for Ethereum Request for Comment 20, which is the technical standard used to create these tokens. ERC-20 tokens are compatible with any Ethereum-based system and can be easily traded, exchanged, or integrated into decentralized applications (dApps).
Creating your own ERC-20 token can serve various purposes, from launching a new cryptocurrency project to creating utility tokens for decentralized applications. In this guide, we will break down how to create an ERC-20 token and what steps you need to take to make it successful.
2. Why Create an ERC-20 Token?
There are several reasons why people create ERC-20 tokens. Here are some common use cases:
Initial Coin Offerings (ICOs): Many companies create ERC-20 tokens to raise funds for their projects through ICOs. These tokens can be sold to investors in exchange for funding.
Utility Tokens: ERC-20 tokens can be used as utility tokens within a platform, granting holders access to specific services, products, or features.
Governance Tokens: ERC-20 tokens can be used in decentralized governance systems, giving token holders voting power over decisions related to the project or platform.
Rewards and Loyalty Programs: Businesses can issue ERC-20 tokens as part of a rewards or loyalty program, incentivizing customer engagement.
By creating your own ERC-20 token, you open up possibilities for interacting with the growing Ethereum ecosystem, giving your project the tools it needs to thrive.
3. Prerequisites for Creating an ERC-20 Token
Before you dive into the technical aspects of creating an ERC-20 token, you need to have a basic understanding of a few key concepts:
A. Understanding Smart Contracts
An Ethereum smart contract is a self-executing contract with the terms of the agreement written directly into code. Smart contracts allow for transactions to take place without the need for intermediaries. They are the backbone of many decentralized applications, including those that involve ERC-20 tokens.
ERC-20 tokens are built using smart contracts on the Ethereum blockchain. You will write a smart contract to define the token’s rules, such as its total supply, transfer mechanisms, and other core functionalities.
B. Basic Knowledge of Solidity
To write a smart contract for an ERC-20 token, you will need to know Solidity, the programming language used to develop smart contracts on the Ethereum network. Solidity is a contract-oriented programming language that is similar to JavaScript but designed for writing smart contracts.
While Solidity is relatively easy to learn for developers with prior experience in JavaScript or other object-oriented languages, it is important to have a grasp of the basics before you start coding your token’s smart contract.
4. Step-by-Step Guide to Creating an ERC-20 Token
Now that you understand the basics, let’s walk through the process of creating your own ERC-20 token step-by-step.
A. Setting Up the Development Environment
To get started, you'll need to set up a development environment for writing and testing your smart contract. Here are the steps:
Install Node.js and NPM: These are essential for running JavaScript-based tools like Truffle, which helps with smart contract development and deployment.
Install Truffle: Truffle is a popular framework for developing Ethereum-based applications. You can install it via npm: npm install -g truffle
Install Ganache: Ganache is a personal Ethereum blockchain used for testing smart contracts.
Set Up MetaMask: MetaMask is a browser extension that allows you to interact with the Ethereum blockchain. You will use it to manage your tokens once deployed.
B. Writing the Smart Contract
Once your environment is set up, it’s time to write the code for your ERC-20 token. Below is a basic example of a Solidity contract for an ERC-20 token:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/token/ERC20/ERC20.sol";
contract MyToken is ERC20 {
constructor(uint256 initialSupply) ERC20("MyToken", "MTK") {
_mint(msg.sender, initialSupply);
}
}
ERC20("MyToken", "MTK"): This defines the name ("MyToken") and symbol ("MTK") of your token.
_mint(msg.sender, initialSupply): This creates an initial supply of tokens and assigns them to your wallet address (msg.sender).
You can adjust the supply and other parameters according to your project’s needs.
C. Deploying the Smart Contract
Once the smart contract is written, it’s time to deploy it to the Ethereum network:
Compile the Contract: Use Truffle to compile the smart contract: truffle compile
Deploy the Contract: Next, deploy the contract using Truffle or directly via Remix (an online Solidity IDE). For deployment on a test network like Rinkeby or Ropsten, use Truffle’s migration tool: truffle migrate --network rinkeby
After deployment, your ERC-20 token is live and can be interacted with.
5. ERC-20 Token Functions Explained
An ERC-20 token includes several standard functions that define its behavior. Here’s a breakdown of the most important ones:
totalSupply(): Returns the total supply of tokens.
balanceOf(address account): Returns the token balance of a specific address.
transfer(address recipient, uint256 amount): Allows a user to transfer tokens to another address.
approve(address spender, uint256 amount): Allows a user to approve another address to spend tokens on their behalf.
transferFrom(address sender, address recipient, uint256 amount): Allows a spender to transfer tokens on behalf of the sender.
allowance(address owner, address spender): Returns the amount of tokens that a spender is allowed to transfer on behalf of the owner.
6. How to Interact with Your ERC-20 Token
After deploying your ERC-20 token, you can interact with it through your MetaMask wallet or dApps:
Adding Your Token to MetaMask: Once your token is deployed, you can add it to MetaMask by using the token's contract address. This will show your token’s balance in MetaMask.
Sending Tokens: You can send tokens to others by using the transfer() function through your wallet interface or dApp.
7. Best Practices for Creating a Secure ERC-20 Token
When creating your ERC-20 token, security is paramount. Here are some best practices to keep in mind:
Auditing Your Code: Always have your smart contract code audited by professionals to check for vulnerabilities or flaws.
Test Thoroughly: Before deploying to the main network, test your contract on a test network (like Rinkeby or Kovan) to ensure it works as expected.
Limit Token Minting: Avoid creating an unlimited supply of tokens. Consider implementing a cap to prevent inflation and ensure that the token’s value remains stable.
Use Reputable Libraries: Leverage open-source and well-established libraries like OpenZeppelin for ERC-20 token implementation to avoid common coding errors.
8. Token Audits and Security Considerations
Security audits are crucial to ensuring your ERC-20 token is safe from potential attacks. A smart contract audit involves a thorough review of the code to identify vulnerabilities, including:
Reentrancy attacks
Overflow/Underflow issues
Gas limit issues
You can hire third-party services that specialize in auditing smart contracts or use automated tools to scan your code.
9. Real-World Applications of ERC-20 Tokens
ERC-20 tokens have found numerous real-world applications:
DeFi: ERC-20 tokens are widely used in decentralized finance platforms like lending, borrowing, and yield farming.
Gaming: Many blockchain-based games use ERC-20 tokens to represent in-game assets, rewards, or currencies.
NFTs: ERC-20 tokens can be used alongside ERC-721 or ERC-1155 tokens to create unique collectible items in digital markets.
10. Conclusion: Start Your Token Journey Today
Creating an ERC-20 Token: A Beginner’s Guide. Creating your own ERC-20 token is an exciting and powerful way to participate in the rapidly growing world of decentralized applications and cryptocurrency. Whether you’re planning to launch a new ICO, create a governance token, or build an ecosystem for your project, ERC-20 tokens provide the flexibility, security, and accessibility needed to succeed.
FAQ Creating an ERC-20 Token: A Beginner’s Guide
Q1: Do I need a centralized exchange to trade ERC-20 tokens?
No, ERC-20 tokens can be traded on decentralized exchanges (DEXs) such as Uniswap or Sushiswap.
Q2: How much does it cost to create an ERC-20 token?
The cost of creating an ERC-20 token depends on deployment fees, which are generally paid in Ethereum (ETH) to cover gas costs. Fees vary depending on network congestion.
Q3: Can I change the token's parameters after deployment?
Once deployed, an ERC-20 token's parameters cannot be changed. If needed, a new contract would need to be deployed.
Q4: What is the difference between ERC-20 and ERC-721 tokens?
ERC-20 tokens are fungible, meaning each token is identical. ERC-721 tokens, on the other hand, are non-fungible and are typically used for unique digital items like NFTs.
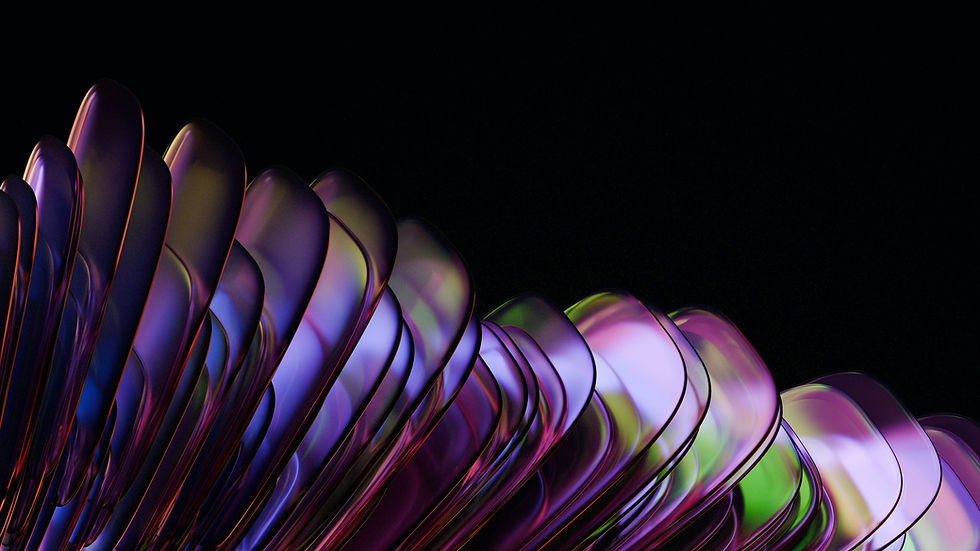